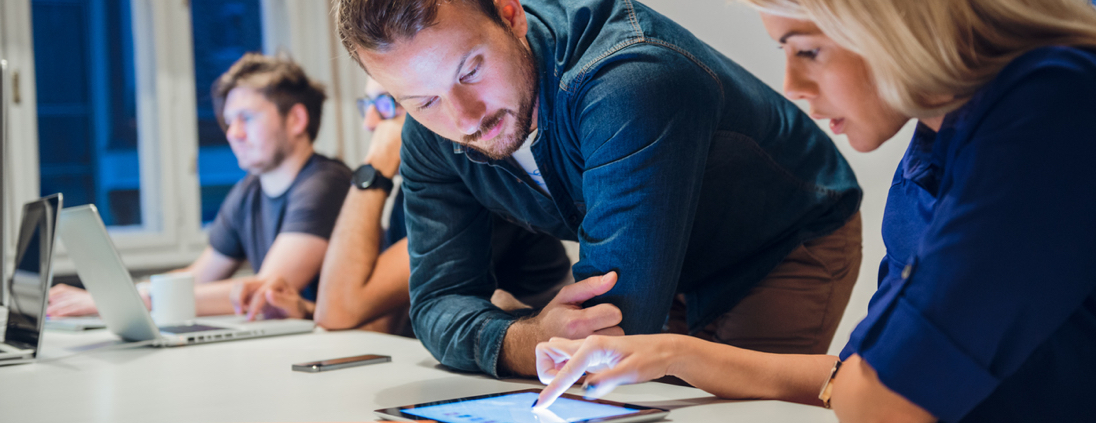
Nanodegree key: nd003
Version: 4.0.0
Locale: en-us
Master writing in Swift as you build five portfolio-worthy iOS apps to demonstrate your expertise as an iOS Developer.
Content
Part 01 : Welcome to the Nanodegree
-
Module 01: Welcome to the Nanodegree
-
Lesson 01: Get Help with Your Account
What to do if you have questions about your account or general questions about the program.
-
Lesson 02: Nanodegree Career Services
The Careers team at Udacity is here to help you move forward in your career - whether it's finding a new job, exploring a new career path, or applying new skills to your current job.
-
Lesson 03: Get Ready to Learn iOS
Welcome to the iOS Nanodegree! This is where your journey to become an iOS developer begins. Learn about the structure of the program, and determine the best path for you.
-
Part 02 : Learn Swift Programming
Learn the basics of Swift, the programming language used to develop iOS apps.
-
Module 01: Learn Swift Programming
-
Lesson 01: Variables and Types
Learn about one of the most basic building blocks of nearly every programming language. Variables give developers the ability to store information that can be used to control the behavior.
- Concept 01: Welcome
- Concept 02: Interlude: Download Xcode
- Concept 03: Course Sandbox
- Concept 04: Exercise: Your First Lines of Swift
- Concept 05: Exercise: Hello World
- Concept 06: Exercise Review
- Concept 07: Intro to Variables
- Concept 08: Data Types
- Concept 09: Creating Variables
- Concept 10: Type Inference
- Concept 11: Changing a Variable’s Value
- Concept 12: Constants
- Concept 13: Naming Variables and Constants
- Concept 14: Tips With String Literals
- Concept 15: Tips With String Literals (Continued)
- Concept 16: Lesson 1 Conclusion
-
Lesson 02: Operators and Expressions
Add, subtract, multiply, divide, and more using operators and expressions in Swift!
- Concept 01: Introduction to Operators and Expressions
- Concept 02: Operators in Playgrounds
- Concept 03: Terms to Remember
- Concept 04: Working With Operator Expressions
- Concept 05: Back to Game Points
- Concept 06: Looking Ahead at New Operators
- Concept 07: Boolean Expressions
- Concept 08: Equalities and Comparisons
- Concept 09: The AND Operator
- Concept 10: The OR Operator
- Concept 11: The NOT Operator
-
Lesson 03: Control Flow
Learn how to control when and how Swift code is executed by using if and else statements.
- Concept 01: Intro to If Statements
- Concept 02: If Statements
- Concept 03: If Statements Practice
- Concept 04: If Else and Else If Statements
- Concept 05: If, Else If Practice
- Concept 06: More on Conditionals
- Concept 07: Switch Statements
- Concept 08: Loops
- Concept 09: For Each Loops
- Concept 10: While Loops
- Concept 11: The Break Keyword
- Concept 12: Repeat While
-
Lesson 04: Functions
Learn how to define and use functions, which give developers the ability to bundle up code so that it can be reused multiple times.
-
Lesson 05: Structures and Enums
Learn how to declare and use structures, or structs, in Swift, and explore Enums, a type that can represent any value in a set of related values.
-
Lesson 06: Optionals
Why does Swift need this data type that no other language has? Join us as we get to the bottom of this mystery, and learn optionals inside and out.
-
Lesson 07: Strings
Playing with Strings is a lot of fun. In this lesson, we'll explore Swift’s String struct, and practice some common string manipulations.
-
Lesson 08: Collections
It’s time to explore collection types, including Arrays and Dictionaries.
-
Lesson 09: Object-Oriented Programming
Learn foundational concepts of object oriented programming, including classes, inheritance, and polymorphism.
-
Part 03 : Intro to iOS App Development with Swift
Build your first app with Swift and Xcode, Apple’s programming environment for app development.
-
Module 01: Intro to iOS App Development with Swift
-
Lesson 01: Introduction and Xcode
An introduction to Swift and Xcode. Swift is Apple's fast-growing programming language for building iOS apps and much more. Xcode is the programming environment used to create native Apple applications with Swift.
- Concept 01: Introduction
- Concept 02: Course Map
- Concept 03: Pitch Perfect Overview
- Concept 04: Creating a Hello World Swift iOS App
- Concept 05: Tour of Xcode
- Concept 06: Document Outline in Xcode
- Concept 07: Xcode Shortcuts
- Concept 08: Introduction to the MVC Pattern
- Concept 09: MVC Pattern in Mail.app
- Concept 10: What's Next
-
Lesson 02: AutoLayout and Buttons
Learn about Storyboards — Xcode's interface design tool. Use AutoLayout, UIButtons, and UILabels to create an interface. Start developing the views for the Pitch Perfect app.
- Concept 01: Introduction
- Concept 02: Intro to the Storyboard
- Concept 03: AutoLayout Basics
- Concept 04: AutoLayout Minimum Info Needed
- Concept 05: Quiz on AutoLayout Constraints
- Concept 06: Constraints to the Record Button
- Concept 07: Alternative Way to Create Constraints
- Concept 08: Adding Code for the Button
- Concept 09: From Code to UI Element
- Concept 10: Note on IBOutlets, IBActions, and Constraints
- Concept 11: Difference Between IBAction and IBOutlet
- Concept 12: Connecting the Stop Recording Button
- Concept 13: Adding Constraints for the New Buttons
- Concept 14: More Info on AutoLayout
- Concept 15: Where Do Buttons Come From
-
Lesson 03: ViewController and Multiple Views
Learn how to react to touch events and change the user interface accordingly.
- Concept 01: Introduction
- Concept 02: Application Lifecycle
- Concept 03: Opening Documentation in Xcode
- Concept 04: UIViewController viewDidLoad
- Concept 05: ViewDidLoad and Friends
- Concept 06: Setting State Before a View Appears
- Concept 07: Enable and Disable the Recording Buttons
- Concept 08: Adding Images to the Recording Buttons
- Concept 09: Resizing the Stop Button
- Concept 10: Disabling the Stop Recording Button
- Concept 11: How to Enable or Disable a UI Button
- Concept 12: Enabling and Disabling the Buttons
- Concept 13: Multiple Views
- Concept 14: Using a UINavigation Controller
- Concept 15: Adding a Second View Controller
- Concept 16: UINavigationController
- Concept 17: Recap and Next Steps
- Concept 18: Recap What You Have Learned
-
Lesson 04: Delegation and Recording
Introduction to AVAudioRecorder, Delegation, and programmatic segues. Setup audio recording in the Pitch Perfect App
- Concept 01: Lesson Overview
- Concept 02: AVAudioRecorder and Deciphering New Code
- Concept 03: AVAudioRecorder Code Explanation
- Concept 04: Renaming the Recording View Controller
- Concept 05: Adding the AVAudioRecorder
- Concept 06: Recording Issues
- Concept 07: Fixing the Segue
- Concept 08: The Meaning of the Word Delegate
- Concept 09: Adding Delegation to RecordSoundsVC
- Concept 10: Summarize What You Learned (Delegation)
- Concept 11: Sending the Recorded Audio File
- Concept 12: Creating the PlaySoundsViewController
- Concept 13: Setup the PlaySoundsViewController
- Concept 14: Prepare for Segue and Receiving the Data
- Concept 15: Wrapup and What's Ahead
-
Lesson 05: Playback and Audio Effects
Setup audio playback including rate, pitch, echo, and reverb. Learn about UIStackViews and class extensions.
- Concept 01: Lesson Overview
- Concept 02: StackViews as a Concept
- Concept 03: Inserting StackViews
- Concept 04: Snail and Rabbit Button Icons
- Concept 05: StackView for Pitch Effects
- Concept 06: StackViews for Echo+Reverb and Stopping Playback
- Concept 07: Fixing the UI
- Concept 08: Wiring up Buttons (IBOutlets+IBActions)
- Concept 09: Troubleshooting IBOutlets and IBActions
- Concept 10: Adding the Audio Extension
- Concept 11: Playing Back Audio
- Concept 12: PlaySoundsViewController Extension
- Concept 13: Clean Up
- Concept 14: Wrap Up
- Concept 15: Reflection
-
-
Module 02: Brainstorm Ideas
-
Lesson 01: Brainstorm Ideas for Your Final App!
Get a kickstart on your open-ended final app by brainstorming potential ideas!
- Concept 01: Welcome to "Project" 1.5!
- Concept 02: First iOS Apps by Experienced Developers
- Concept 03: Let's Build an App!
- Concept 04: Benefits of Logging your Progress
- Concept 05: Course Map and Mindset
- Concept 06: What Makes a "Good" iOS App?
- Concept 07: Starting at the Drawing Board
- Concept 08: Brainstorm Ideas: Personal Motivation
- Concept 09: What Inspires or Frustrates You?
- Concept 10: Brainstorm Ideas: Featured Apps
- Concept 11: Ideas from Featured Apps
- Concept 12: Lacking Ideas? Let's Get Creative!
- Concept 13: Advice for Getting Creative
- Concept 14: Get Feedback on your Ideas!
- Concept 15: How Did Users React?
-
-
Module 03: Project: Pitch Perfect
-
Lesson 01: Pitch Perfect
Create an iPhone app that records a conversation with you and a friend and plays it back to make you sound like a chipmunk or Darth Vader!
-
-
Module 04: Suggested Electives
-
Lesson 01: Suggested Electives
Learn how to store your code on Github, and review Swift syntax if needed, before proceeding.
-
Part 04 (Elective): Version Control with Git
Learn how to use git, a popular version control system and essential tool for any developer.
-
Module 01: Version Control with Git
-
Lesson 01: What is Version Control?
Version control is an incredibly important part of a professional programmer's life. In this lesson, you'll learn about the benefits of version control and install the version control tool Git!
-
Lesson 02: Create A Git Repo
Now that you've learned the benefits of Version Control and gotten Git installed, it's time you learn how to create a repository.
-
Lesson 03: Review a Repo's History
Knowing how to review an existing Git repository's history of commits is extremely important. You'll learn how to do just that in this lesson.
-
Lesson 04: Add Commits To A Repo
A repository is nothing without commits. In this lesson, you'll learn how to make commits, write descriptive commit messages, and verify the changes you're about to save to the repository.
-
Lesson 05: Tagging, Branching, and Merging
Being able to work on your project in isolation from other changes will multiply your productivity. You'll learn how to do this isolated development with Git's branches.
-
Lesson 06: Undoing Changes
Help! Disaster has struck! You don't have to worry, though, because your project is tracked in version control! You'll learn how to undo and modify changes that have been saved to the repository.
-
Part 05 (Elective): GitHub and Collaboration
Learn how to interact with remote repositories, and collaborate with other developers on GitHub.
-
Module 01: Github & Collaboration
-
Lesson 01: Working With Remotes
You'll learn how to create remote repositories on GitHub and how to get and send changes to the remote repository.
-
Lesson 02: Working On Another Developer's Repository
In this lesson, you'll learn how to fork another developer's project. Collaborating with other developers can be a tricky process, so you'll learn how to contribute to a public project.
-
Lesson 03: Staying In Sync With A Remote Repository
You'll learn how to send suggested changes to another developer by using pull requests. You'll also learn how to use the powerful
git rebase
command to squash commits together.
-
Part 06 : UIKit Fundamentals
Develop an app with UIKit, Apple’s front-end framework for developing fast and powerful web interfaces.
-
Module 01: UIKit Fundamentals: Part 1
-
Lesson 01: Outlets and Actions
Connect outlets and actions using only code and graphically using storyboard. Use the following UIKit classes: UIButton, UILabel, UISwitch.
- Concept 01: Introduction
- Concept 02: MemeMe App
- Concept 03: Course Map
- Concept 04: Click Counter and its Object Diagram
- Concept 05: Coding Click Counter
- Concept 06: Click Counter Quiz
- Concept 07: Creating the Count and Label Properties
- Concept 08: Diagramming the target action
- Concept 09: Setting the Target Action
- Concept 10: Experimenting with Target Action
- Concept 11: Actions as Callback Methods
- Concept 12: Transitioning to Storyboard Label
- Concept 13: Transitioning to Storyboard, Button
- Concept 14: Actions & Outlets Quiz
- Concept 15: Intro: Switches for the Color Maker App
- Concept 16: Researching Switches for Color Maker
- Concept 17: Diagramming Color Maker
- Concept 18: Connecting the Color Maker
- Concept 19: Help with Connecting the Color Maker
- Concept 20: Debugging Color Maker Error 1
- Concept 21: Debugging Color Maker Error 2
- Concept 22: Debugging Color Maker Error 3
- Concept 23: Challenge App: Color Maker with Sliders
- Concept 24: Challenge App Quiz
- Concept 25: End of Lesson 1
-
Lesson 02: View Presentations and Segues
Learn how to present views modally using the following UIKit classes: UIImagePickerController, UIAlertController, UIActivityViewController.
- Concept 01: Presenting View Controllers
- Concept 02: Get Your Game Face On!
- Concept 03: Modal Presentation vs Navigation
- Concept 04: How does a view get presented modally?
- Concept 05: Launch Image Picker and Activity View
- Concept 06: Launch Activity View and Alert View
- Concept 07: Summarize code for 3 Experiments
- Concept 08: Dice Example, Take Control
- Concept 09: Presenting the Dice View Controller
- Concept 10: Presenting View Controllers: Code Only
- Concept 11: How Do ViewControllers Communicate?
- Concept 12: Demonstrate Code & Segue
- Concept 13: Code & Segue Review
- Concept 14: Segue Only
- Concept 15: Steps For Segue Only
- Concept 16: Passing Data Between ViewControllers
- Concept 17: prepareForSegue
- Concept 18: Review of prepareForSegue
- Concept 19: Challenge App: Roshambo
- Concept 20: Steps to Build Roshambo
- Concept 21: Challenge App Quiz
- Concept 22: Lesson 2 End
-
Lesson 03: The Delegate Pattern
Most common UIKit components make use of the delegate pattern. Learn how delegates make important connections between the model, view, and controller.
- Concept 01: Introducing Delegates
- Concept 02: Reuse with Customizaton
- Concept 03: The Course Github Repository
- Concept 04: Compare and Contrast Custom Text Fields
- Concept 05: Introducing Protocols
- Concept 06: Real World Examples of Protocols
- Concept 07: Text Field Code Review
- Concept 08: The UITextFieldDelegate Protocol
- Concept 09: Diagramming the Text Field App
- Concept 10: A Look at the Other Two Delegates
- Concept 11: Creating a Random Color Delegate
- Concept 12: Random Color Delegate Code
- Concept 13: Preparing for the Challenge Apps
- Concept 14: Challenge Apps
- Concept 15: Challenge App Quiz
- Concept 16: Next Up: MemeMe v1.0
-
Lesson 04: Build V1.0 of the MemeMe App
Create a first version of the MemeMe app that enables a user to take a picture, add text, and share the created meme on Facebook, Twitter, SMS, or email.
- Concept 01: MemeMe 1.0 Project Intro
- Concept 02: MemeMe 1.0 Demo
- Concept 03: MemeMe 1.0 To-Do List
- Concept 04: Reflecting on the To-Do List
- Concept 05: Picking Images
- Concept 06: Researching the UIImagePicker Delegate
- Concept 07: Receiving an Image Using a Delegate
- Concept 08: What About the Camera?
- Concept 09: Textfield Specifications
- Concept 10: Working with Text Attributes
- Concept 11: Move View Get Out the Way
- Concept 12: Code for Keyboard Adjustments
- Concept 13: Implementing "keyboardWillHide"
- Concept 14: Recommended Reading on NSNotifications
- Concept 15: Generating a Meme Object
- Concept 16: Sharing a Meme using an Activity View
-
-
Module 02: Project: MemeMe 1.0
-
Lesson 01: MemeMe 1.0
You'll create a first version of the MemeMe app that enables a user to take a picture, and add text at the top and bottom to form a meme.
-
-
Module 03: UIKit Fundamentals: Part 2
-
Lesson 01: Table Views
Table views are one of the most commonly used views in iOS apps. Learn how to implement tables in your own apps.
- Concept 01: Recalling Text Field Delegate
- Concept 02: Protocol Methods Review
- Concept 03: Observing HealthKit Safari and Contacts
- Concept 04: Guessing the Properties of Data Struct
- Concept 05: Key Delegate & Data Source Questions
- Concept 06: Recognizing Method Signatures
- Concept 07: Favorite Things
- Concept 08: How cellForRowAtIndexPath Wrangles Cells
- Concept 09: Implementing cellForRowAtIndexPath
- Concept 10: Do-Re-Mi App
- Concept 11: Approaching the Problem from Storyboard
- Concept 12: Exploring the Bond Villains Project
- Concept 13: Setting up Bond Villians in Storyboard
- Concept 14: Connecting the Storyboard in Xcode
- Concept 15: Choosing Cell Styles
- Concept 16: Challenge App: Roshambo with History
- Concept 17: Steps to Build Roshambo with History
- Concept 18: Challenge App Quiz
- Concept 19: Next Up: Navigation
-
Lesson 02: Navigation
Learn how iOS uses navigation stacks to manage multiple views in an app.
- Concept 01: Recalling Navigation
- Concept 02: Navigation in Your Favorite Apps
- Concept 03: Make Your Own Adventure: The Easy Way
- Concept 04: MYOA Steps and Solution
- Concept 05: Adding Start Over Button: Step 1
- Concept 06: Adding Start Over Button: Step 2
- Concept 07: Start Over Button Steps and Solution
- Concept 08: Navigation and the Stack Data Structure
- Concept 09: How Can We Verify the Stack Behavior
- Concept 10: Intro to Rock Paper Scissors
- Concept 11: Replacing RPS Logic with Navigation
- Concept 12: RPS with Navigation - Solution
- Concept 13: Master-Detail, Navigation for Tables
- Concept 14: Bond Villians Using Master Detail
- Concept 15: Steps to Rebuild BondVillains
- Concept 16: Challenge App: Data-Driven MYOA
- Concept 17: Notes on the Challenge App
- Concept 18: Challenge App Quiz
- Concept 19: Congrats
-
Lesson 03: Complete the MemeMe App
Memes will appear in a tab view with two tabs: a table view and a collection view.
- Concept 01: MemeMe 2.0 Project Intro
- Concept 02: MemeMe 2.0 Demo
- Concept 03: MemeMe 2.0 To-Do List
- Concept 04: Using a Shared Model
- Concept 05: Code for Using a Shared Model
- Concept 06: Example Code: Tabs & Collection Views
- Concept 07: TabViewControllers: Villains in Tabs
- Concept 08: Setup the Sent Memes Collection View
- Concept 09: Setup a Collection View in Storyboard
- Concept 10: Recap: Set up the CollectionView in SB
- Concept 11: Setting up a Collection View Flow Layout
- Concept 12: Code for Collection View Flow Layout
- Concept 13: Troubleshooting Collection Views
- Concept 14: Way to go!
-
-
Module 04: Project: MemeMe 2.0
-
Lesson 01: Project: MemeMe 2.0
You'll create a final version of the MemeMe App. Memes will appear in a tab view with two tabs: a table view and a collection view.
-
Part 07 (Elective): AutoLayout
Learn about AutoLayout, and how to use stack views and constraints to create pixel-perfect UIs.
-
Module 01: AutoLayout
-
Lesson 01: Introduction to AutoLayout
In this lesson, you will learn what problems AutoLayout solves and why you can't afford to ignore it any longer.
-
Lesson 02: Using AutoLayout
In this lesson, you will learn about the 4 different ways of implementing AutoLayout, which one we recommend you use, and why. You also will delve deeper into the recommended way: UIStackViews.
-
Lesson 03: View Properties used by AutoLayout
AutoLayout uses 3 properties that all views share: intrinsicContentSize, compressionResistence and contentHugging. Don't be put away by the weird names! It's actually pretty simple, and in this lesson, you'll learn all about them.
-
Lesson 04: Beginning StackViews
In this lesson, you will learn about 4 properties of StackViews that define its apparently weird behavior. Once you're done with this lesson, you will understand there's a logic to StackViews, and a lot of power too.
-
Lesson 05: Positioning Views & Constraints
In this lesson, your will learn what a constraint is and well as the role of constraints in AutoLayout.
- Concept 01: What Does It Mean To Position A View?
- Concept 02: Sufficient Constraints
- Concept 03: Creating a Row of Views
- Concept 04: Anatomy of a Constraint
- Concept 05: Different Devices
- Concept 06: Expand to Take the Whole Width
- Concept 07: Content Hugging and Compression Resistance
- Concept 08: Compression Resistance and Content Hugging Revisited
- Concept 09: Content Hugging Put to Test
- Concept 10: Of Margins and Training Wheels
-
Lesson 06: Horizontal Layouts
In this lesson, we will create simple horizontal layouts. These will be building blocks for more complex layouts.
-
Lesson 07: Vertical Layouts
In this lesson, we will take composition and vertical layouts to the next step and use them to create more complex layouts.
-
Lesson 08: Beyond AutoLayout
Before you leave, let's take a look at what you went through and what might be lying ahead.
-
Part 08 (Elective): Sketch UI Elements
Sketch UI elements for your final app, and start visualizing what it might look like.
-
Module 01: Sketch UI Elements
-
Lesson 01: Sketch UI Elements for Your Final App!
Now that you know a bit more about UI elements available to you, sketch out some potential UIs for your final app ideas!
- Concept 01: Welcome to "Project" 2.5!
- Concept 02: On Developing Multiple Ideas
- Concept 03: UI Elements for App Ideas
- Concept 04: Table Views or Collection Views?
- Concept 05: Navigation or Modal Presentation?
- Concept 06: Anything You're Unable to Implement?
- Concept 07: Post Sketches for Your App Ideas
-
Part 09 : Network Requests and GCD
Incorporate networking into your apps, and harness the power of APIs to display images and retrieve data. Use Apple’s Grand Central Dispatch, or GCD, framework to create asynchronous apps, ensuring a smooth user experience, even while your apps run lengthy operations in the background.
-
Module 01: Introduction
-
Lesson 01: Welcome to Part 2!
So far, you've focused largely on UIKit and various UI elements of your apps. Now, you'll learn how to deal with network requests and storing data to the device.
-
-
Module 02: Swift for Networking
-
Lesson 01: Prereq: Associated Values
Associated values are values that can be attached to enum cases. In this lesson, learn how to use associated values to enhance enums while simultaneously improving their readability and conciseness.
-
Lesson 02: Prereq: Guards
Guards, or guard statements, specify conditions that must be true for execution to continue. By using guards, you can create preconditions and safely control execution.
-
Lesson 03: Prereq: Errors
In this lesson, learn how to handle errors in Swift. These errors, unlike warnings or issues raised by the compiler, are generated in situations where correct behavior cannot be guaranteed.
- Concept 01: Intro
- Concept 02: Handle Errors
- Concept 03: Types of Errors
- Concept 04: Handle Errors
- Concept 05: Propagate Errors
- Concept 06: Quiz: Propagate Errors
- Concept 07: The Many Faces of Try
- Concept 08: The Many Faces of Try
- Concept 09: Error Handling Summary
- Concept 10: Displaying Errors to Users
- Concept 11: Create Custom Errors
- Concept 12: Quiz: Create Custom Errors
- Concept 13: Describe Custom Errors
- Concept 14: Cleanup with Defer
- Concept 15: Outro
- Concept 16: Exercises
-
Lesson 04: Prereq: Generics
Generics is one of the most powerful features in Swift. It powers Swift arrays, dictionaries, and collections, and it can be used to apply functionality to specified types.
-
Lesson 05: Prereq: Closures Reloaded
Learn about closures, an alternative syntax to functions, as well as how
typealias
allows you to rename existing types.- Concept 01: Closures Reloaded
- Concept 02: What Makes Closures So Special
- Concept 03: Flying First Class
- Concept 04: First Class Demo
- Concept 05: The Answer to Life...
- Concept 06: Adding Closures to an Array
- Concept 07: Same Old Functions and Closures
- Concept 08: Functions and Closures
- Concept 09: Variable Capture Intro
- Concept 10: Variable Capture and Type Alias
- Concept 11: Type Alias Example
- Concept 12: Variable Capture at Last
- Concept 13: Type Alias
- Concept 14: Capture
- Concept 15: Outro
-
-
Module 03: iOS Networking with Swift
-
Lesson 01: Networking Foundations: HTTP
See what happens behind the scenes when you visit a website and learn the basics of HTTP networking.
- Concept 01: Intro
- Concept 02: Interview with Kaya Thomas: Getting Started
- Concept 03: How Apps Get Data
- Concept 04: Postman
- Concept 05: Using Postman
- Concept 06: Anatomy of a URL
- Concept 07: Status Codes
- Concept 08: HTTP Verbs
- Concept 09: HTTP Request and Response
- Concept 10: HTTPS
- Concept 11: Interview with Kaya Thomas: The Next Steps
- Concept 12: Back to Swift!
-
Lesson 02: Networking Foundations: Swift
Apply your knowledge of HTTP networking to make GET requests in Swift and use GCD to ensure a responsive UI.
- Concept 01: Intro
- Concept 02: URL
- Concept 03: URLComponents
- Concept 04: Storing URLComponents
- Concept 05: URLSession and Data Tasks
- Concept 06: Making Network Requests
- Concept 07: Interview with Kaya Thomas: HTTPS
- Concept 08: App Transport Security
- Concept 09: Multithreading and the Main Thread
- Concept 10: Changing Data Task to Download Task
- Concept 11: Summary
-
Lesson 03: Intro to Web Services
Visit the documentation for a third party web API and use it to build a networked iOS app.
- Concept 01: Intro
- Concept 02: The Dog API
- Concept 03: Interview with Kaya Thomas: APIs and Web Services
- Concept 04: An App Idea
- Concept 05: Starting the Project
- Concept 06: Storing the Endpoint
- Concept 07: Making the Request
- Concept 08: What is JSON?
- Concept 09: Parsing JSON with JSONSerialization
- Concept 10: Interview with Kaya Thomas: Codable
- Concept 11: Introducing the Codable Protocol
- Concept 12: From JSON to Struct with Codable
- Concept 13: Fetching the Image
- Concept 14: A Quick Detour on Closures
- Concept 15: Chaining Requests Refactor Part 1
- Concept 16: Chaining Requests Refactor Solution
- Concept 17: Summary
-
Lesson 04: Consuming Data
Get practice parsing JSON and learn a variety of parsing techniques using Swift's Codable protocol.
- Concept 01: Intro
- Concept 02: JSON Parsing Review
- Concept 03: A New Feature
- Concept 04: Breed List Response
- Concept 05: Coding Keys
- Concept 06: Coding Keys Walkthrough
- Concept 07: JSON Arrays
- Concept 08: Arrays Walkthrough
- Concept 09: Nested JSON Objects
- Concept 10: Nested Objects Walkthrough
- Concept 11: Accessing Keys and Values Directly
- Concept 12: Breeds List Setup
- Concept 13: Filter By Breed
- Concept 14: Populate the Breeds List
- Concept 15: Breeds List Walkthrough
- Concept 16: Interview with Kaya Thomas: JSON Parsing
- Concept 17: Summary
-
Lesson 05: Authentication
Learn about the authentication process, access user-specific data, and make your first POST request in Swift.
- Concept 01: Intro: The Movie Manager
- Concept 02: Interview with Travis Bell: The Movie Database
- Concept 03: TMDB Setup
- Concept 04: API Keys
- Concept 05: Movie Manager Tour
- Concept 06: Interview with Travis Bell: Auth Flow
- Concept 07: GET The Request Token
- Concept 08: POST Requests in Swift
- Concept 09: Login with Email And Password
- Concept 10: Create Session ID
- Concept 11: Interview with Travis Bell: Another Auth Question
- Concept 12: Working with URLs in iOS Apps
- Concept 13: OAuth-like Authentication in the Movie Manager
- Concept 14: Logging out
- Concept 15: DELETEing a Session
- Concept 16: Summary
-
Lesson 06: Deeper API Interaction
Practice reading documentation as you add features to a networked app.
- Concept 01: Intro
- Concept 02: Interview with Travis Bell: Favorite Apps
- Concept 03: Improving the Networking Architecture
- Concept 04: Refactoring: taskForGETRequest
- Concept 05: Finish Refactoring taskForPOSTRequest
- Concept 06: Feature: Favorites List
- Concept 07: Feature: Search Movies
- Concept 08: Feature: Add to Watchlist
- Concept 09: Add to Watchlist
- Concept 10: Feature: Add to Favorites
- Concept 11: Add to Favorites
- Concept 12: Feature: Poster Image on Detail View
- Concept 13: Feature: Poster Images in Watchlist
- Concept 14: Summary
-
Lesson 07: Common Networking Challenges
Learn techniques for debugging networked apps, limiting network usage, and properly handling errors.
- Concept 01: Intro
- Concept 02: Movie Manager Code Review
- Concept 03: Code Review: Placeholder Images
- Concept 04: Code Review: Show Network Activity
- Concept 05: Enable and Disable UI Appropriately
- Concept 06: Code Review: Wasted Network Calls
- Concept 07: Code Review: Handling Errors
- Concept 08: Alert for login failure
- Concept 09: Your Turn: Improve the Movie Manager
- Concept 10: Summary: Get Out and Network!
-
-
Module 04: Project On the Map
-
Lesson 01: On the Map
In this project, you’ll pull in data from a web service to display a map with pins. Tapping on a pin will display a custom URL posted by another iOSND student at that location.
- Concept 01: Demo: "On the Map"
- Concept 02: APIs and New Methods
- Concept 03: Making Requests in a Playground
- Concept 04: Parse API: What is a Student Location?
- Concept 05: Parse API: GETting Student Locations
- Concept 06: Parse API: POSTing a Student Location
- Concept 07: Parse API: PUTting a Student Location
- Concept 08: Using the Udacity API
- Concept 09: Udacity API: POSTing a Session
- Concept 10: Udacity API: DELETEing a Session
- Concept 11: Udacity API: GETting Public User Data
- Concept 12: Working with Maps
- Concept 13: On the Map
-
-
Module 05: Career Services
-
Lesson 01: Take 30 Min to Improve your LinkedIn
Find your next job or connect with industry peers on LinkedIn. Ensure your profile attracts relevant leads that will grow your professional network.
- Concept 01: Get Opportunities with LinkedIn
- Concept 02: Use Your Story to Stand Out
- Concept 03: Why Use an Elevator Pitch
- Concept 04: Create Your Elevator Pitch
- Concept 05: Use Your Elevator Pitch on LinkedIn
- Concept 06: Create Your Profile With SEO In Mind
- Concept 07: Profile Essentials
- Concept 08: Work Experiences & Accomplishments
- Concept 09: Build and Strengthen Your Network
- Concept 10: Reaching Out on LinkedIn
- Concept 11: Boost Your Visibility
- Concept 12: Up Next
-
Lesson 02: Optimize Your GitHub Profile
Other professionals are collaborating on GitHub and growing their network. Submit your profile to ensure your profile is on par with leaders in your field.
- Concept 01: Prove Your Skills With GitHub
- Concept 02: Introduction
- Concept 03: GitHub profile important items
- Concept 04: Good GitHub repository
- Concept 05: Interview with Art - Part 1
- Concept 06: Identify fixes for example “bad” profile
- Concept 07: Quick Fixes #1
- Concept 08: Quick Fixes #2
- Concept 09: Writing READMEs with Walter
- Concept 10: Interview with Art - Part 2
- Concept 11: Commit messages best practices
- Concept 12: Reflect on your commit messages
- Concept 13: Participating in open source projects
- Concept 14: Interview with Art - Part 3
- Concept 15: Participating in open source projects 2
- Concept 16: Starring interesting repositories
- Concept 17: Next Steps
-
-
Module 06: Suggested Electives
-
Lesson 01: Suggested Electives
Time to get some more practice with web APIs, and get really comfortable debugging!
-
Part 10 (Elective): Find Web APIs
Find Web APIs to use for your final app.
-
Module 01: Find Web APIs
-
Lesson 01: Find Web APIs for Your Final App
Begin researching potential web APIs to use in your final app.
- Concept 01: Welcome to "Project" 3.5!
- Concept 02: Find Existing APIs and Libraries
- Concept 03: Advice for Choosing Web APIs & Libraries
- Concept 04: Find & Vet Web APIs
- Concept 05: Constructing URLs
- Concept 06: Structure of the Web API's Data
- Concept 07: User Authentication
- Concept 08: Summary and What's Next
-
Part 11 (Elective): iOS Debugging
Learn how to debug your apps quickly and efficiently so that you can boost your productivity as a developer.
-
Module 01: iOS Debugging
-
Lesson 01: Debugging, Printing, and Logging
Meet the "So Many Bugs" app, which is used to solve coding mysteries. Learn about the debugging process, and how to use print statements to identify bugs.
- Concept 01: We Need to Talk About the Bug Problem
- Concept 02: Notice: Code Examples and Repositories
- Concept 03: SoManyBugs: Repository
- Concept 04: SoManyBugs: Demo
- Concept 05: Shake the Bugs
- Concept 06: Warnings, Errors, and Runtime Errors
- Concept 07: Debugging Lingo
- Concept 08: The Debugging Process
- Concept 09: Print Debugging
- Concept 10: Printable and DebugPrintable
- Concept 11: No More Crash!
- Concept 12: Advanced Print Debugging: Logging
- Concept 13: Bonus Debugging Tool: dump
-
Lesson 02: Stepping Through Code
Learn how to use breakpoints to examine application state at any point during execution.
- Concept 01: Breakpoint Debugging
- Concept 02: Setting Breakpoints
- Concept 03: Pausing Our Execution and the Debug Area
- Concept 04: Examining a Stack Frame
- Concept 05: Jumping Around Frames
- Concept 06: The Debug Bar
- Concept 07: Step In, Step Out, Step Over
- Concept 08: Fixing Settings Bug with Breakpoints
- Concept 09: Stack Traces for Creating a Bug
- Concept 10: Debug Navigator Icons
- Concept 11: Variable Kinds
- Concept 12: Getting Help with Bugs
- Concept 13: Good Debuggers are Good Investigators
-
Lesson 03: LLDB and Breakpoint Actions
Learn about LLDB (low-level debugger)—the debugging super tool. Learn common LLDB commands, tips, and tricks.
- Concept 01: Better Debugging with Better Tools
- Concept 02: The Low Level Debugger
- Concept 03: Common LLDB Commands: Part 1
- Concept 04: Common LLDB Commands: Part 2
- Concept 05: Practicing with LLDB
- Concept 06: Practicing with LLDB: Demo
- Concept 07: LLDB Reference Material
- Concept 08: Breakpoint Actions
- Concept 09: Playing with Breakpoint Options
-
Lesson 04: Breakpoints and Visual Tools
Learn about exception and symbolic breakpoints, and how to debug your code using Xcode's visual debugging tools.
- Concept 01: Exception Breakpoints
- Concept 02: Symbolic Breakpoints
- Concept 03: Pro Trip: "Property" Breakpoints
- Concept 04: Fix the Breakpoint Scene
- Concept 05: Quick Look
- Concept 06: Debug View Hierarchy
- Concept 07: Fixing the Visual Bugs
- Concept 08: Fixing the Visual Bugs: Solution Pt. 2
- Concept 09: Xcode Debugging Hotkeys
- Concept 10: Becoming a Debugging Ninja
- Concept 11: Squash All the Bugs!
-
Part 12 : Data Persistence
Learn about simple persistence, the iOS File System, and the “sandbox.” Set up the classes we need to get Core Data up and running so that we can create, save, and delete model objects. Enable user interfaces to reactively update whenever the model changes, and safely migrate user data between versions.
-
Module 01: Grand Central Dispatch
-
Lesson 01: GCD and Queues
Understand how and what tasks you can run in the background on an app and what must always run in the foreground.
- Concept 01: GCD and Queues
- Concept 02: GCD and Threads
- Concept 03: Types of Queues
- Concept 04: Serial Queues: Can I Have Concurrency?
- Concept 05: Main Functions in GCD
- Concept 06: Dispatch Async
- Concept 07: Execution Order
- Concept 08: Beware of UIKit and Core Data!
- Concept 09: Will it Crash: The Toughest Bugs Ever
- Concept 10: Outro
-
Lesson 02: Backgrounding Lengthy Tasks
Create a simple app that downloads huge images. Apply your newly acquired knowledge to send this network lengthy task to the background in two different ways.
-
-
Module 02: iOS Persistence and Core Data
-
Lesson 01: Simple Persistence
Learn about simple persistence and how to save small pieces of data, like user preference, using NSUserDefaults.
- Concept 01: Intro
- Concept 02: Course Overview
- Concept 03: UserDefaults Intro
- Concept 04: When Should You Use UserDefaults?
- Concept 05: Example Before We Dive into the Code
- Concept 06: Pick Your Pitch
- Concept 07: Adding Persistence to Pick Your Pitch
- Concept 08: Finishing Pick Your Pitch
- Concept 09: Outro
-
Lesson 02: iOS File System and Sandboxing
Learn about the iOS File System, the “sandbox”, and how to access these files using NSFileManager.
-
Lesson 03: Introducing Core Data
Explore what a data layer is, and how to convert a non-Core Data app to have a Core Data model.
- Concept 01: Introduction
- Concept 02: About Data Layers
- Concept 03: What is Core Data?
- Concept 04: More than Persistence
- Concept 05: Why Persist Data?
- Concept 06: Why Apps Close
- Concept 07: Persistence Options
- Concept 08: Introducing Mooskine
- Concept 09: Downloading Mooskine
- Concept 10: The Existing Model
- Concept 11: Adding a Core Data Model
- Concept 12: Entities
- Concept 13: Attributes
- Concept 14: Attribute Types
- Concept 15: Optional Attributes
- Concept 16: Adding Attributes to Note
- Concept 17: Relationships
- Concept 18: Two-Sided Relationships and Deletion Rules
- Concept 19: Entities Review
- Concept 20: Code Generation
- Concept 21: Codegen Consequences
- Concept 22: Summary
-
Lesson 04: The Core Data Stack
Set up the classes we need to get Core Data up and running so that we can create, save, and delete model objects.
- Concept 01: Introduction
- Concept 02: The Core Data Stack
- Concept 03: Stack Facts
- Concept 04: Setting Up the Stack in Mooskine
- Concept 05: Switching to Code-Generated Classes
- Concept 06: Injecting the DataController Dependency
- Concept 07: Fetching Data for NotebooksListViewController
- Concept 08: Adding and Deleting Notebooks
- Concept 09: Fetch Requests, Faults and Uniquing
- Concept 10: Creating and Saving Managed Objects
- Concept 11: Making a Fetch Request
- Concept 12: Practice: Adapt NotesListViewController
- Concept 13: Updating NotesListViewController
- Concept 14: NSPredicate
- Concept 15: A Predicate Predicament
- Concept 16: Saving Edits in NoteDetailsViewController
- Concept 17: When Should You Save?
- Concept 18: Subclassing in Core Data
- Concept 19: Context Concerns
- Concept 20: Extending Model Classes
- Concept 21: Customizing Note Initialization
- Concept 22: Singletons and Dependency Injection
- Concept 23: Review
-
Lesson 05: Simpler Code with Core Data
Enable user interfaces to reactively update whenever the model changes.
- Concept 01: Introduction
- Concept 02: Refactoring
- Concept 03: Complexity in Mooskine
- Concept 04: NSFetchedResultsController
- Concept 05: Core Data Notifications
- Concept 06: Adding a Fetched Results Controller
- Concept 07: Fetched Results Controller and Collections
- Concept 08: Displaying Data
- Concept 09: Tracking Changes
- Concept 10: Adding Change Types
- Concept 11: Number of Rows in a Section
- Concept 12: NSFetchedResultsControllerDelegate
- Concept 13: Caching
- Concept 14: Practice: NotesListViewController
- Concept 15: Generic Data Source
- Concept 16: Review
-
Lesson 06: Rounding Out Core Data
Safely migrate user data between versions, and move slow work to the background.
- Concept 01: Competitive Analysis
- Concept 02: Introduction
- Concept 03: Storing Rich Text with NSAttributedString
- Concept 04: Binary and transformable attributes
- Concept 05: Changing a Data Model
- Concept 06: Adding a New Data Model
- Concept 07: Migration Types
- Concept 08: Custom mapping models
- Concept 09: Creating a new model version
- Concept 10: Transformable
- Concept 11: Preparing the keyboard
- Concept 12: Pathifier Helper
- Concept 13: Adding Text Formatting
- Concept 14: Concurrency in Core Data
- Concept 15: Context Architecture Choices
- Concept 16: Context Creation and Keeping Queues Straight
- Concept 17: Context Merge Policies
- Concept 18: Creating a Background Context
- Concept 19: Using a Background Context
- Concept 20: Observing Notifications
- Concept 21: Running Mooskine
- Concept 22: Concurrency in Core Data
- Concept 23: Summary
-
-
Module 03: Project: Virtual Tourist
-
Lesson 01: Project: Virtual Tourist
Build an app that lets you tour the world from the comfort of your home. Users will be able to drop pins on a map, download pictures for the location, and save favorites to their device.
-
-
Module 04: Suggested Elective
-
Lesson 01: Optional Elective: Firebase in a Weekend - Saturday
Learn about additional data persistence solutions to impress employers! We highly encourage you to do this elective. It is optional.
- Concept 01: Optional Elective Info
- Concept 02: Firebase in a Weekend!
- Concept 03: Overview
- Concept 04: Favorite Features
- Concept 05: Examination of Final App
- Concept 06: Download the Friendly Chat App
- Concept 07: Firing Up FriendlyChat
- Concept 08: Firebase in Xcode
- Concept 09: Installing Cocoapods
- Concept 10: Setting up Cocoapods for FriendlyChat
- Concept 11: Starter Code Walkthrough
- Concept 12: Starter Code Walkthrough Exercise
- Concept 13: Firebase Realtime Database
- Concept 14: Why Firebase Realtime Database?
- Concept 15: Database Structure
- Concept 16: Exploring the Database
- Concept 17: Sending a Message
- Concept 18: Database Rules for Testing
- Concept 19: Reading from the Database
- Concept 20: Reading Messages
- Concept 21: Showing Messages
- Concept 22: Database Rules for Testing
- Concept 23: Database Security
- Concept 24: Database Security Rules
- Concept 25: Advanced Database Rules
- Concept 26: Why is it Great to Authenticate?
- Concept 27: Why is it Great to Authenticate? (Exercise)
- Concept 28: FirebaseUI Authentication
- Concept 29: Authentication in Firebase Console
- Concept 30: Getting Started with FirebaseUI
- Concept 31: Configuring Email and Password Authentication
- Concept 32: Configuring Google Authentication
- Concept 33: Saturday Check In
-
Lesson 02: Optional Elective: Firebase in a Weekend - Sunday
During the Sunday lesson, you'll be getting into more advanced topics. They include:
- Using Firebase Storage to allow users to upload picture messages from their phone
- Securing your user's uploade
- Concept 01: Sunday Funday
- Concept 02: Firebase Storage Features
- Concept 03: Creating Storage Structure
- Concept 04: Sending Friendly Photos
- Concept 05: Message in a Bottle
- Concept 06: A Fix and a Break
- Concept 07: Message Received!
- Concept 08: Bonus: Seeing the Bigger Picture
- Concept 09: Keep Your Stuff Safe
- Concept 10: Not Your Parents' Permissions
- Concept 11: Playing Matchmaker
- Concept 12: Playing Matchmaker Exercise
- Concept 13: Follow the Rules
- Concept 14: Contexts or Chaos
- Concept 15: Reading in Context
- Concept 16: Securing it All Together
- Concept 17: Analytics Answer “Now What?”
- Concept 18: Analyze This
- Concept 19: Notify Me
- Concept 20: Notifications Next Steps
- Concept 21: Remote Config: Little Changes, Big Impact
- Concept 22: Policies and Limits
- Concept 23: Talking Too Much
- Concept 24: Fixing Message Length
- Concept 25: Remote Config - Conclusion
- Concept 26: Making Firebase Your Homebase
- Concept 27: Conclusion/Extra Practice
-
Lesson 03: Optional Elective: Firebase in a Weekend - Monday
Got extra time this weekend? As a bonus, you will write your own Cloud Function for Firebase that makes chat more fun by adding emojis to FriendlyChat conversations. Cloud Functions for Firebase integ
- Concept 01: Intro to Monday Module
- Concept 02: Swift to JavaScript Comparison
- Concept 03: Intro to Cloud Functions
- Concept 04: Install the Firebase CLI
- Concept 05: Create the Local Project
- Concept 06: Cloud Functions Structure
- Concept 07: Function with Realtime Database
- Concept 08: Deploy and Test
- Concept 09: Emojify in FriendlyChat App
- Concept 10: More Functions
- Concept 11: Cloud Functions Conclusion
-
Part 13 (Elective): Firebase in a Weekend
This course will teach you when and why to choose Firebase as a backend for your iOS application.
-
Module 01: Firebase in a Weekend
-
Lesson 01: Firebase in a Weekend: Saturday
Start the weekend off right by implementing Firebase in FriendlyChat. FriendlyChat is a realtime messaging application. In this lesson you will:
- Create a Firebase project in the Firebase console
- Setup Firebase in FriendlyChat
- Read and write chat message data to the Firebase Realtime Database
- Secure your database with Firebase's security rules language
- Setup login and authentication for users
- Concept 01: Weekend Plans
- Concept 02: Course Contents
- Concept 03: Favorite Features
- Concept 04: Examination of Final App
- Concept 05: Creating a Firebase Project
- Concept 06: Download the Friendly Chat App
- Concept 07: Firing Up FriendlyChat
- Concept 08: Firebase in Xcode
- Concept 09: Installing Cocoapods
- Concept 10: Setting up Cocoapods for FriendlyChat
- Concept 11: Starter Code Walkthrough
- Concept 12: Starter Code Walkthrough Exercise
- Concept 13: Firebase Realtime Database
- Concept 14: Why Firebase Realtime Database?
- Concept 15: Database Structure
- Concept 16: Exploring the Database
- Concept 17: Configuring the Database in FriendlyChat
- Concept 18: Sending a Message
- Concept 19: Database Rules for Testing
- Concept 20: Reading from the Database
- Concept 21: Reading Messages
- Concept 22: Showing Messages
- Concept 23: Database Rules for Testing
- Concept 24: Database Security
- Concept 25: Database Security Rules
- Concept 26: Advanced Database Rules
- Concept 27: Why is it Great to Authenticate?
- Concept 28: Why is it Great to Authenticate? (Exercise)
- Concept 29: FirebaseUI Authentication
- Concept 30: Authentication in Firebase Console
- Concept 31: Getting Started with FirebaseUI
- Concept 32: Configuring Email and Password Authentication
- Concept 33: Configuring Google Authentication
- Concept 34: Saturday Check In
-
Lesson 02: Firebase in a Weekend: Sunday
During the Sunday lesson, you'll be getting into more advanced topics. They include:
- Using Firebase Storage to allow users to upload picture messages from their phone
- Securing your user's uploaded files using Firebase's Storage rules
- Accessing and analyzing your application's data about user behaviors
- Sending notifications to users from the Firebase console
- Using Firebase Remote Config to test a new max length for FriendlyChat messages, all without an app update
- Concept 01: Sunday Funday
- Concept 02: Firebase Storage Features
- Concept 03: Creating Storage Structure
- Concept 04: Sending Friendly Photos
- Concept 05: Message in a Bottle
- Concept 06: A Fix and a Break
- Concept 07: Message Received!
- Concept 08: Bonus: Seeing the Bigger Picture
- Concept 09: Keep Your Stuff Safe
- Concept 10: Not Your Parents' Permissions
- Concept 11: Playing Matchmaker
- Concept 12: Playing Matchmaker Exercise
- Concept 13: Follow the Rules
- Concept 14: Contexts or Chaos
- Concept 15: Reading in Context
- Concept 16: Securing it All Together
- Concept 17: Analytics Answer “Now What?”
- Concept 18: Analyze This
- Concept 19: Notify Me
- Concept 20: Notifications Next Steps
- Concept 21: Remote Config: Little Changes, Big Impact
- Concept 22: Policies and Limits
- Concept 23: Talking Too Much
- Concept 24: Fixing Message Length
- Concept 25: Remote Config - Conclusion
- Concept 26: Making Firebase Your Homebase
- Concept 27: Conclusion/Extra Practice
-
Lesson 03: Firebase in a Weekend: Monday
Got extra time this weekend? As a bonus, you will write your own Cloud Function for Firebase that makes chat more fun by adding emojis to FriendlyChat conversations. Cloud Functions for Firebase integrates the Firebase platform by letting you write code that responds to events and invokes functionality exposed by other Firebase features.
- Concept 01: Intro to Monday Module
- Concept 02: Swift to JavaScript Comparison
- Concept 03: Intro to Cloud Functions
- Concept 04: Install the Firebase CLI
- Concept 05: Create the Local Project
- Concept 06: Cloud Functions Structure
- Concept 07: Function with Realtime Database
- Concept 08: Deploy and Test
- Concept 09: Emojify in FriendlyChat App
- Concept 10: More Functions
- Concept 11: Cloud Functions Conclusion
-
Part 14 (Elective): Firebase Analytics
Learn how to grow an app's user base using Firebase Analytics, a free and unlimited analytics solution. You'll be able to learn who your users are and how they interact with your app.
-
Module 01: Firebase Analytics
-
Lesson 01: Introduction to Digital Analytics
In this lesson, we will take on the role of the developer for a live app: Flood It! We will discuss the foundations of digital analytics using Flood It as a model.
- Concept 01: How Does Your Garden Grow?
- Concept 02: Course Outline
- Concept 03: Analytics: Let the Data Drive
- Concept 04: Check Out Flood-It!
- Concept 05: Download Flood-It!
- Concept 06: Demo of Flood-It!
- Concept 07: Measurement Planning
- Concept 08: Goal of Measurement Planning
- Concept 09: Identifying Goals
- Concept 10: Go for the Goals
- Concept 11: The Plan
- Concept 12: Meet Goals with In-App Purchases
- Concept 13: Planning
- Concept 14: Planning - Conclusion
- Concept 15: The Plan for Your Goals
- Concept 16: Are We There Yet?
- Concept 17: KPI
- Concept 18: KPI for Flood-It!
- Concept 19: Analytics in Action
-
Lesson 02: Introduction to Firebase Analytics
Learn about the Firebase Analytics Dashboard and how to view the data collected by your apps. Also, learn how to build audiences and funnels by leveraging events with Firebase Analytics.
- Concept 01: Intro: Flood-It!
- Concept 02: Firebase Analytics Intro
- Concept 03: Analytics for Flood-It!
- Concept 04: Access Demo Project
- Concept 05: Date Range and Filters
- Concept 06: Quiz: Date Range and Filters
- Concept 07: Dashboard Reports
- Concept 08: Exploring Analytics Events
- Concept 09: Quiz: Events Scavenger Hunt
- Concept 10: Tracking Events
- Concept 11: Automatically Collected Events
- Concept 12: Conversion Events
- Concept 13: Conversion Events in Flood It
- Concept 14: Audiences
- Concept 15: Funnels
- Concept 16: Design a Funnel
- Concept 17: Summary: Flood-It!
-
Lesson 03: Implementing In-App Analytics
Learn how to use built-in events provided by Firebase Analytics and implement some of your own. Then, see how easy it is to tailor an app experience to segments of users with User Properties.
- Concept 01: Intro: Implementing In-App Analytics
- Concept 02: Introducing the Green Thumb App
- Concept 03: Setting Up Green Thumb
- Concept 04: Quiz: Running the App
- Concept 05: Firebase Automatically Collected Events
- Concept 06: Suggested Events
- Concept 07: Quiz: Suggested Events
- Concept 08: Logging Events
- Concept 09: Quiz: Implementing Log to Cart Event
- Concept 10: More Logging Events
- Concept 11: Quiz: Creating the sendAddToCart Function
- Concept 12: Quiz: Implementing the Log to Cart Event
- Concept 13: Custom Event Logging
- Concept 14: Quiz: Event Logging Practice
- Concept 15: User Properties
- Concept 16: Setting User Properties
- Concept 17: Configuring User Properties
- Concept 18: Quiz: Logging the User Property
- Concept 19: Summary: Implementing In-App Analytics
-
Lesson 04: Analytics Integration
In this lesson, you'll build upon what you've learned about Firebase Analytics and explore crash reporting, remote configuration, and dynamic links — a suite of powerful tools for improving your apps.
- Concept 01: Intro: Analytics Integration
- Concept 02: Track Certain Groups of Users with Audiences
- Concept 03: Quiz: Create an Audience
- Concept 04: Using an Audience
- Concept 05: Fix Crashes with Crash Reporting
- Concept 06: Configuring Crash Reporting
- Concept 07: Quiz: Report a Crash
- Concept 08: Improve Your App with Remote Config
- Concept 09: Implementing Remote Config
- Concept 10: Dynamic Links
- Concept 11: Implementing Dynamic Links
- Concept 12: Custom Parameters for Dynamic Links
- Concept 13: Quiz: Implementing Dynamic Links
- Concept 14: Summary: Analytics Integration
- Concept 15: Conclusion
-
Part 15 : Project: You Decide!
This is your chance to let your iOS Developer skills shine! For this final project, you'll design and build your own iOS app, taking the design from the drawing board to the App Store.
-
Module 01: How to Make an iOS App
-
Lesson 01: Research
Brainstorm and vet multiple app ideas. Think about the UI elements used in each, get user feedback on designs, and investigate relevant web APIs and libraries.
- Concept 01: First iOS Apps by Experienced Developers
- Concept 02: Let's Build an App!
- Concept 03: Benefits of Logging your Progress
- Concept 04: Course Map and Mindset
- Concept 05: What Makes a "Good" iOS App?
- Concept 06: Starting at the Drawing Board
- Concept 07: Brainstorm Ideas: Personal Motivation
- Concept 08: What Inspires or Frustrates You?
- Concept 09: Brainstorm Ideas: Featured Apps
- Concept 10: Ideas from Featured Apps
- Concept 11: Lacking Ideas? Let's Get Creative!
- Concept 12: Advice for Getting Creative
- Concept 13: Get Feedback on your Ideas!
- Concept 14: How Did Users React?
- Concept 15: On Developing Multiple Ideas
- Concept 16: UI Elements for App Ideas
- Concept 17: Table Views or Collection Views?
- Concept 18: Navigation or Modal Presentation?
- Concept 19: Anything You're Unable to Implement?
- Concept 20: Post Sketches for Your App Ideas
- Concept 21: Find Existing APIs and Libraries
- Concept 22: Advice for Choosing Web APIs & Libraries
- Concept 23: Find & Vet Web APIs
- Concept 24: Constructing URLs
- Concept 25: Structure of the Web API's Data
- Concept 26: User Authentication
- Concept 27: Summary and What's Next
-
Lesson 02: Build
Choose an idea to pursue and build it! We'll share tips for getting started, dealing with code that doesn't work, and how to submit to the App Store.
- Concept 01: It's (Almost) Time to Start Building!
- Concept 02: Define Features for Your App Ideas
- Concept 03: Refine Your Feature Lists
- Concept 04: Choose Key Feature
- Concept 05: It's the Moment of Truth
- Concept 06: How Confident Are You?
- Concept 07: Create a Paper Prototype
- Concept 08: Reminder to Check out UX Design
- Concept 09: Get Input & Refine Your Paper Prototype
- Concept 10: Estimate Time to Completion
- Concept 11: Follow Your Timeline
- Concept 12: Where to Find Great Graphics
- Concept 13: Build Your App
- Concept 14: Tips for Getting Started
- Concept 15: What About When You Get Stuck?
- Concept 16: Help Getting Unstuck
- Concept 17: Share Your Working Prototype!
- Concept 18: Testing 1, 2, 3...
- Concept 19: Get Users to Find Bugs
- Concept 20: Polish and Prep Your App
- Concept 21: App Store: What You'll Need
- Concept 22: App Store: Assets
- Concept 23: App Store: Certificate
- Concept 24: App Store: App ID
- Concept 25: App Store: Provisioning Profile
- Concept 26: App Store: Archive
- Concept 27: App Store: Bringing it All Together
- Concept 28: You did it! Hip-Hip-Hoorah!
-
Lesson 03: Reflect
Reflect on the process: What went well, and what you might do differently next time? Recognizing and learning from mistakes is what makes you a better developer!
-
-
Module 02: Project: You Decide!
-
Lesson 01: Project: You Decide!
This is your chance to let your iOS Developer skills shine! For this final project, you'll design and build your own iOS app, taking the design from the drawing board to the App Store.
-
-
Module 03: Suggested Electives
-
Lesson 01: Suggested Electives
Continue your career progress, and take our Technical Interview Prep and Mobile Design Patterns courses to help ace your next interview.
-
Part 16 (Elective): Objective-C for Swift Developers
This series of coding challenges is designed to prepare you for the most common causes of friction between Objective-C and Swift.
-
Module 01: Objective-C for Swift Developers
-
Lesson 01: Objective-C vs. Swift
Learn the distinguishing language features of Objective-C.
- Concept 01: Introduction
- Concept 02: Welcome to Objective-C for Swift Devs!
- Concept 03: Intro to Dasmer Singh
- Concept 04: Interview with Dasmer Singh
- Concept 05: Compare and Contrast
- Concept 06: Similarities: Objective-C and Swift
- Concept 07: Differences: Handling Nil
- Concept 08: Differences: Mutability
- Concept 09: Mutability in Objective-C
- Concept 10: NSArray vs. NSMutableArray
- Concept 11: Differences: Typing
- Concept 12: In Objective-C Arrays are not Typed
- Concept 13: Dynamic Method Resolution
- Concept 14: The Power Is In Your Hands
- Concept 15: With Great Power...
- Concept 16: Interview Questions About Objective-C
- Concept 17: Practice: NSArray vs. NSMutableArray
- Concept 18: Practice: Invoking Methods on Nil
- Concept 19: Interview Question: Inheritance
- Concept 20: Seek Out More Practice Questions
-
Lesson 02: Writing Classes in Objective-C
Familiarize yourself with Objective-C syntax. Write some classes and properties from scratch.
- Concept 01: Introduction
- Concept 02: Class Declaration
- Concept 03: Code for the House Class
- Concept 04: Property Attributes: nonatomic and atomic
- Concept 05: Readwrite, Readonly, and Copy
- Concept 06: Class Initialization
- Concept 07: Understanding Self
- Concept 08: Code for the Custom Initializer
- Concept 09: Practice: Write the Book Class
- Concept 10: Making Two Classes Work Together
- Concept 11: More Property Attributes: Strong and Weak
- Concept 12: Practice: Build on the Book Project
- Concept 13: Practice: Rock, Paper, Scissors
- Concept 14: Lesson 2 Outro
-
Lesson 03: Methods and Messages in Objective-C
Master how to write and call methods in Objective-C. Build a functioning game.
- Concept 01: Introduction to Lesson 3
- Concept 02: Review Method Definition Syntax
- Concept 03: Practice: Method Definitions
- Concept 04: Calling Methods: Syntax and Vocabulary
- Concept 05: Why Do We Say "Send a Message"?
- Concept 06: Quiz: Methods and Messages
- Concept 07: Add a Game Property
- Concept 08: Finishing the Method throwDown
- Concept 09: Properties: Provide Setters and Getters
- Concept 10: Control Flow: Switch Statements
- Concept 11: Putting the generateMove Method to Work
- Concept 12: Control Flow: If Statements
- Concept 13: Practice: Messaging in Objective-C
- Concept 14: Exercise: RPS Helper Methods
- Concept 15: Making a Method Visible to Other Classes
- Concept 16: Exercise: RPS Results Message
- Concept 17: Exercise: RPS, Putting It All Together
- Concept 18: Nice work! Next Up...
-
Lesson 04: Porting from Objective-C to Swift (Part 1)
Write custom classes and incorporate third party frameworks and extensions.
- Concept 01: Interview with Keith Smiley from Lyft
- Concept 02: Lesson 4 Introduction
- Concept 03: GIFMaker Demo
- Concept 04: Getting Started
- Concept 05: App Components
- Concept 06: To Do List
- Concept 07: Present the Video Camera
- Concept 08: What's Analogous to a Category?
- Concept 09: UIViewController Extension
- Concept 10: Display a GIF in a UIImage
- Concept 11: Implement Regift Methods
- Concept 12: Display the GIF in the GifEditor
- Concept 13: Do You See the New GIF?
- Concept 14: Convert a Video into a GIF? Check!
- Concept 15: Rewriting the Gif Class
- Concept 16: Sample Swift Gif Class
- Concept 17: Configure the Editor to Add a Caption
- Concept 18: UITextFieldDelegate Methods
- Concept 19: UITextFieldDelegate Methods: Sample Code
- Concept 20: Make Room for the Keyboard
- Concept 21: Make Room for the Keyboard: Sample Code
- Concept 22: Add a Caption to the Animated GIF
- Concept 23: What is this Method Doing?
- Concept 24: Let's Walk Through presentPreview
- Concept 25: Add a Caption? Check!
- Concept 26: Share the GIF
- Concept 27: Share the GIF: Sample Code
- Concept 28: Share the GIF? Check!
- Concept 29: Create an Action Sheet
- Concept 30: Convert an Existing Video? Check!
- Concept 31: Enable Video Trimming: Steps
- Concept 32: Enable Video Trimming: Sample Code
- Concept 33: Crop GIF to be Square
- Concept 34: Quiz: How Did the Cropping Go?
- Concept 35: Finishing Touches
- Concept 36: Get Ready for the Next Iteration...
-
Lesson 05: Porting from Objective-C to Swift (Part 2)
Persist data with NSCoder and NSKeyedArchiver. Implement navigation with a collection view.
- Concept 01: Introduction
- Concept 02: GIFMaker 2.0 Demo
- Concept 03: GifMaker 20 Todo List
- Concept 04: Setup the SavedGifsViewController
- Concept 05: UICollectionView Protocols
- Concept 06: Setup the PreviewVC and its Delegate
- Concept 07: View Saved GIFs in a Collection? Check!
- Concept 08: Conform to the NSCoding Protocol
- Concept 09: Sample Code for the NSCoding Protocol
- Concept 10: Handling Untyped Arrays
- Concept 11: Construct a File Path for the GIFs Array
- Concept 12: Sample Code to Create the File Path
- Concept 13: Save and Retrieve the GIFs
- Concept 14: Where Should We Unarchive the GIFs?
- Concept 15: Persist GIFs Check!
- Concept 16: The GIF Detail View
- Concept 17: Sample Code for the GIF Detail View
- Concept 18: First Time User Experience
- Concept 19: Styling
- Concept 20: Add this Project to Your Portfolio
- Concept 21: Well Done! Next Up...
-
Lesson 06: Common Interop Challenges
Facilitate communication between the languages of iOS. Complete a set of coding challenges to sharpen interop skills.
- Concept 01: Remember Dasmer
- Concept 02: Interview with Dasmer Singh
- Concept 03: #1 Nil Values
- Concept 04: Nullability Annotations
- Concept 05: Quiz: Annotate the Book Class
- Concept 06: #2 The Hazards of ID and AnyObject
- Concept 07: Taking Care with AnyObject Optional Chaining and Casting
- Concept 08: Lightweight Generics
- Concept 09: Quiz: ID and the Hazards of AnyObject
- Concept 10: #3 Error Handling
- Concept 11: How Swift Interprets an NSError
- Concept 12: How Objective-C Interprets Swift Errors
- Concept 13: #4 Is Your Swift API visible to Objective-C?
- Concept 14: Using @objc
- Concept 15: Mixed Language Projects and Importing Files
- Concept 16: Outro
- Concept 17: Objective-C Course Finale
-
-
Module 02: Interoperability Problem Set
-
Lesson 01: Interoperability Problem Set
Develop good habits for dealing with variables exported by Objective-C in forms that are difficult for Swift to handle.
-
Part 17 (Elective): Mobile Design Patterns
This course is strongly recommended for all students who want to gain mastery of common techniques—or design patterns—for organizing and structuring code in iOS apps.
-
Module 01: Mobile Design Patterns
-
Lesson 01: Principles of Software Design
Learn the core principles and tenets of software design.
- Concept 01: Introduction to Software Design
- Concept 02: Software Design Principles
- Concept 03: Which Design is Extensible, Encapsulated, and Reusable?
- Concept 04: Keep it DRY
- Concept 05: SOLID Foundations
- Concept 06: Single Responsibility Principle
- Concept 07: Open/Closed Principle
- Concept 08: Liskov Substitution Principle
- Concept 09: Interface Segregation Principle
- Concept 10: Dependency Inversion Principle
- Concept 11: SOLID Exercise
- Concept 12: Summary: Gang of Four Design Patterns
-
Lesson 02: Creational Design Patterns
Understand what creational design patterns are and when to apply them.
- Concept 01: Creational Design Patterns
- Concept 02: Factory Method
- Concept 03: Static Factory Method
- Concept 04: Controlling Access
- Concept 05: Singleton
- Concept 06: When Lazy is Good
- Concept 07: Dependency Injection
- Concept 08: No Dependency Injection
- Concept 09: Dependency Injection vs Dependency Inversion
- Concept 10: Object Pool
- Concept 11: Object Pool Exercise
- Concept 12: Object Pools on iOS
- Concept 13: Builder
- Concept 14: Builder Exercise
- Concept 15: Summary
-
Lesson 03: Structural Design Patterns
Understand what structural design patterns are and when to apply them.
-
Lesson 04: Behavioral Design Patterns
Learn about behavioral design patterns, and know when to apply them.
-
Lesson 05: Software Anti-Patterns
Understand why learning software anti-patterns, and writing code “the wrong way” is just as important as learning best practices when designing scalable and maintainable code.
- Concept 01: Introduction to Software Anti-Patterns
- Concept 02: Code Smell
- Concept 03: God Object
- Concept 04: God Object Exercise
- Concept 05: God Object Exercise: Solution
- Concept 06: Deep Inheritance
- Concept 07: Deep Inheritance Exercise
- Concept 08: Global Variables
- Concept 09: The Takeaway
- Concept 10: Congratulations!
-
Part 18 (Elective): Technical Interview Prep
Get hands-on practice and detailed walk-throughs of data structures and algorithms. Note that the programming interface in this course is still in beta, and is continually being improved.
-
Module 01: Technical Interviewing Prep - Swift
-
Lesson 01: Please Read Before Taking this Course
A reminder that the Swift Workspace, your programming interface in this course, is still in beta and is continually being improved.
-
Lesson 02: Introduction and Efficiency
Start off with a basic introduction to topics covered in this course and the overall content structure, including an explanation of expected Swift knowledge.
- Concept 01: Course Introduction
- Concept 02: Course Outline
- Concept 03: Course Expectations
- Concept 04: Syntax
- Concept 05: Swift Syntax Review
- Concept 06: Swift Practice
- Concept 07: Efficiency
- Concept 08: Notation Intro
- Concept 09: Notation Continued
- Concept 10: Worst Case and Approximation
- Concept 11: Efficiency Practice
-
Lesson 03: List-Based Collections
Learn the definition of a list in computer science, and see definitions and examples of list-based data structures, arrays, linked lists, stacks, and queues.
- Concept 01: Welcome to Collections
- Concept 02: Lists
- Concept 03: Arrays
- Concept 04: Swift Arrays
- Concept 05: Linked Lists
- Concept 06: Linked Lists in Depth
- Concept 07: Linked Lists in Swift
- Concept 08: Linked Lists Practice
- Concept 09: Linked Lists Walkthrough
- Concept 10: Stacks
- Concept 11: Stacks Details
- Concept 12: Stacks in Swift
- Concept 13: Stacks Walkthrough
- Concept 14: Queues
- Concept 15: Queues in Swift
- Concept 16: Queues Walkthrough
-
Lesson 04: Searching and Sorting
Explore how to search and sort with list-based data structures, including binary search and bubble, merge, and quick sort. Learn how to use recursion.
- Concept 01: Binary Search
- Concept 02: Efficiency of Binary Search
- Concept 03: Binary Search in Swift
- Concept 04: Binary Search Walkthrough
- Concept 05: Recursion
- Concept 06: Recursion in Swift
- Concept 07: Recursion Walkthrough
- Concept 08: Intro to Sorting
- Concept 09: Bubble Sort
- Concept 10: Efficiency of Bubble Sort
- Concept 11: Bubble Sort Practice
- Concept 12: Merge Sort
- Concept 13: Efficiency of Merge Sort
- Concept 14: Merge Sort Practice
- Concept 15: Quick Sort
- Concept 16: Efficiency of Quick Sort
- Concept 17: Quick Sort in Swift
- Concept 18: Quick Sort Walkthrough
-
Lesson 05: Maps and Hashing
Understand the concepts of sets, maps (dictionaries), and hashing. Examine common problems and approaches to hashing, and practice with examples.
- Concept 01: Introduction to Maps
- Concept 02: Sets and Maps
- Concept 03: Swift Dictionaries
- Concept 04: Dictionaries Walkthrough
- Concept 05: Introduction to Hashing
- Concept 06: Hashing
- Concept 07: Collisions
- Concept 08: Load Factor
- Concept 09: Hash Maps
- Concept 10: String Keys
- Concept 11: String Keys in Swift
- Concept 12: String Keys Walkthrough
-
Lesson 06: Trees
Learn the concepts and terminology associated with tree data structures. Investigate tree types, such as binary search trees, heaps, and self-balancing trees.
- Concept 01: Trees
- Concept 02: Tree Basics
- Concept 03: Tree Terminology
- Concept 04: Tree Practice
- Concept 05: Tree Traversal
- Concept 06: Depth-First Traversals
- Concept 07: Tree Traversal Practice
- Concept 08: Search and Delete
- Concept 09: Insert
- Concept 10: Binary Tree in Swift
- Concept 11: Binary Tree Walkthrough
- Concept 12: Binary Search Trees
- Concept 13: BSTs
- Concept 14: BST Complications
- Concept 15: BST in Swift
- Concept 16: BST Walkthrough
- Concept 17: Heaps
- Concept 18: Heapify
- Concept 19: Heap Implementation
- Concept 20: Self-Balancing Trees
- Concept 21: Red-Black Trees - Insertion
- Concept 22: Tree Rotations
-
Lesson 07: Graphs
Examine the theoretical concept of a graph and understand common graph terms, coded representations, properties, traversals, and paths.
- Concept 01: Graph Introduction
- Concept 02: What Is a Graph?
- Concept 03: Directions and Cycles
- Concept 04: Connectivity
- Concept 05: Graph Practice
- Concept 06: Graph Representations
- Concept 07: Adjacency Matrices
- Concept 08: Graphs in Swift
- Concept 09: Graph Representation Practice
- Concept 10: Graph Representation Walkthrough
- Concept 11: Graph Traversal
- Concept 12: DFS
- Concept 13: BFS
- Concept 14: Graph Traversal Practice
- Concept 15: Graph Traversal Walkthrough
- Concept 16: Eulerian Path
-
Lesson 08: Case Studies in Algorithms
Explore famous computer science problems, specifically the Shortest Path Problem, the Knapsack Problem, and the Traveling Salesman Problem.
-
Lesson 09: Technical Interviewing Techniques
Learn about the “algorithm” for answering common technical interviewing questions. Practice and get tips for giving interviewers what they’re looking for.
- Concept 01: Interview Introduction
- Concept 02: Clarifying the Question
- Concept 03: Confirming Inputs
- Concept 04: Test Cases
- Concept 05: Brainstorming
- Concept 06: Runtime Analysis
- Concept 07: Coding
- Concept 08: Coding 2
- Concept 09: Debugging
- Concept 10: Interview Wrap-Up
- Concept 11: Time for Live Practice with Pramp
- Concept 12: Next Steps
-